INFO PAGE
Programming Basics
This page explores programming basics that are common for most languages. We are using JavaScript, one of the most popular programming languages and traditionally the programming language in Web Browsers.
JavaScript in an Object Oriented Language that differs from some others in that data types are not required. TypeScript can be used to add types to JavaScript.
STATEMENTS
Scripting is basically instructions for the computer to do something. Each instruction ends in a semicolon (optional) and is called a statement. Statements are like steps in the instructions and generally run consecutively. Using a // means ignore the line - we call this a comment and the text goes grey. /* many lines of comments go here */ allows you to do a multiple line comment.
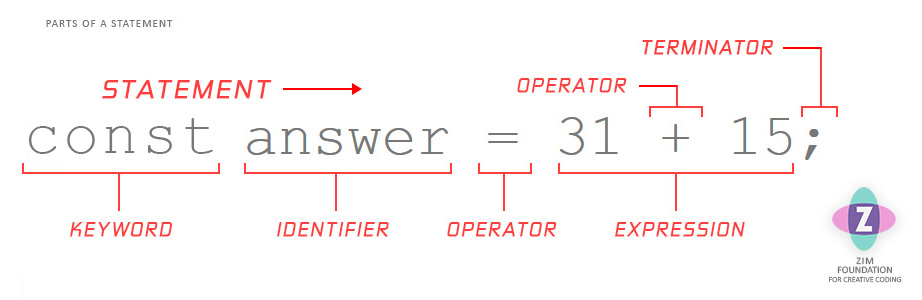
PARTS OF A STATEMENT
Within a statement, there are different parts - words and special characters. These might be keywords belonging to the language (const, for, if, function) or they could be operators - again belonging to the language (+,-,>,!). They could be identifiers that we have made up to label objects (myVar, winner) and they could be objects like numbers, strings, dates, etc. (7, "hello").
You may hear the term Expression. An expression is anything that has a value. "Hello" is an expression, x + 5 is an expression (operand operator operand). This is all getting a little too specific for me so let's carry on...
BLOCKS
We can group statements between TWO curly braces {} and call it a block of code. Sometimes, in our list of statements, we run or call blocks of code. These execute (run) and then we go back to our original list of statements. This means that the overall code does not necessarily run from top to bottom. Examples of this are Functions where we call a block of code whenever needed and Loops where we repeat a block of code over and over for efficiencies. We often even nest blocks of code within blocks of code {{}}. But, within blocks of code, statements proceed from top to bottom ;-)
Each block of code should be indented. This is optional but helps keep the code organized. Think of each block between brackets as a box. It makes coding much easier to keep things organized. Note that VS Code will match brackets when selected, color code matching brackets and draw lines down for indents.
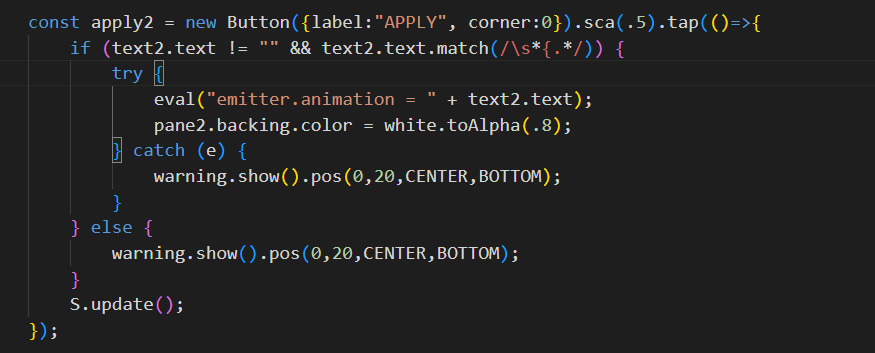
SYNTAX
When we code, there are only certain terms we can use and in certain order. This is called the Syntax of the language. If you use the wrong commands or in the wrong way, you will get an error. The error will show up in the command console. (CTRL SHIFT I or F12 - to toggle the console) If code coloring is turned on (as it is in VS Code) you should see (colors differ for the code in this doc):
- keywords in blue
- comments in green
- classes, methods, properties in white
- number objects in faint green
- string objects in orange
- operators in white
- brackets in pink, blue, yellow to match each other
- identifiers in white
- html parameters in light blue
OBJECTS
Object Oriented Programming (OOP) is a great big important topic. Objects are made from Classes which have the code to make an object. We use the new keyword new to make an object.
new Date();
Objects are the things that we see or work with - like objects in life. They have properties like: location, color, text, rotation. They have methods - things you can do with them or to them: play, open. There are events that can happen to the object: click, complete, mouseover. Pretty well everything that is not a keyword is an object. The browser window is an object, the document is an object, a div is an object. We can make our own objects - see Object Oriented Basics document.
LOGIC
Aside from syntax and objects, there is what to do with the objects. This is where we apply the rules of the environment and is indeed programming. There are only a half dozen types of statements that we use over and over. Below are the parts that help us program - the world's greatest puzzle!
PROGRAMMING CONSTRUCTS
1. Variables
a holder, a container with a label. Here we use the keyword let to declare a variable with an identifier x We then assign the number 7 to the variable x using the assignment operator:let x; x = 7; let y = 9; // or we usually declare and assign in one statement console.log(y); // would show 9 in the console (F12) find CONSOLE
If we know the value will always be the same object then we can use const.
const c = "hello"; console.log(c + " Dr Abstract"); // concatenate the strings together c = "goodbye"; // error as c cannot have a different object
In older JavaScript we use the keyword var for both const and let.
var old = "can still be used"; old = "but we do not use"; // okay as it is like let
2. Conditionals
Tests to compare the value of an expression. Here we use the if else keywords round brackets and curly brackets. We use the comparison operator == to test if x is equal to 7. We use the strict comparison operator === to test if the are equal and the same data type. We run the first block of code if the x == 7 Boolean expression is true. We run the second block of code if the x == 7 Boolean expression is false. These blocks of code could have many statements.if (x == 7) { // do this; } else { // do this; } // there is also an if () {} else if () {} else {} // there is also !=, >, >= , <, <=, === (tests for same type) const date = new Date(); if (date) { // no need for (date == true) // if a date is made } if (!date) { // no need for (date == false) // if date object is null or undefined or 0 or "" }
3. Functions
Declares a block of code to be run any time the function is called. Here we declare a function with an identifier of test and collect a parameter n. We define a block of code that does a conditional test on n. If the test is true we return true and otherwise we return false. return is a keyword that makes the function end and send back a value.- We can make functions without parameters
- We can make functions that do not return a value
- It depends on what we want the function to do
function test(n) { // we indent the block of code to make it easier to read // as we start nesting code, indenting is very important if (n * 5 > 50) {return true;} // {} on single line is optional return false; } test(x);
Functions can be called anywhere in the code as many times as we want. We can even call the function before we define it. JavaScript will "hoist" the declarations and definitions to the top automatically.
Anonymous Function
We can make an anonymous functions:const f = function() {console.log("x is = " + x);} f(); // if x exists then it would concatenate to display its value.
Arrow Function
We can make arrow functions - often used inside event calls:const f = ()=>{console.log("x is = " + x);} f();
Arrow functions have different configurations so can be quite handy.
4. Scope
Scope is where a variable or function can be accessed. In general, a var or a function can be accessed inside the {} of the function block it is held within. If it is not in any function then it is global in scope. The const and let variables are scoped to within any {} block of code including a function {} but also inside if {} and loop {}.const a = 10; function do() { console.log(a); // 10 const b = 20; } console.log(b); // error as b is declared in do {} if (a > 5) { let c = 10; } c += 5; // error as c is declared in if {}
5. Loops
A loop repeats a block of code while a condition is true. Here we loop starting with a variable i having a value of 0. We keep looping as long as i is less than 10. Each time we finish a loop we add one to the value of i. The i++ is a short form for i=i+1. Each time we loop we do what is in the block of code. So we would execute (call) the function makeMonster ten times:for (let i=0; i<10; i++) { makeMonster(); }
There are other types of loops as well such as for(let item in obj), while(), array.forEach(item=>{})
6. Arrays
An array is a list of variables each with an index that increases from zero.const a = ["Dan", "Andrew", "Wasim", "Janice"]; a[0]; // refers to the first element in the array "Dan" console.log(a[1]); // displays "Andrew" in the log window a[0] = "Dan Zen"; // assigns "Dan Zen" to first element of array // it is okay to change inside the array even though we use const // that is because it is still the same array object stored in a // Arrays can be nested - any number of levels (multidimensional arrays): const nest = [["A","B"], ["C","D"]]; console.log(nest[1][0]); // C
Arrays have push(), pop(), unshift(), and shift() methods to add or remove from the end and add or remove from the start. There are splice(), slice(), sort(), reverse(), forEach() methods and more. And a single lenght property!
7. Objects
An object literal {} is a collection of properties each with a value that we access via identifier.const o = {name:"Dan Zen", occupation:"Inventor"} o["name"]; // has a value of "Dan Zen" o.name; // is the same thing and has a value of "Dan Zen" o["occupation"] = "Professor"; // replaces "Inventor" with "Professor" o.occupation = "Inventor"; // puts "Inventor" back into occupation // it is okay to change object properties even though we use const // that is because it is still the same object stored in o // objects can hold any property including arrays: const mixed = {parts:["engine", "wheel"]}; console.log(mixed.parts[1]); // wheel // arrays can hold objects: const family = [{name:"Harold"}, {name:"Jess"}]; console.log(family[0].name); // Harold